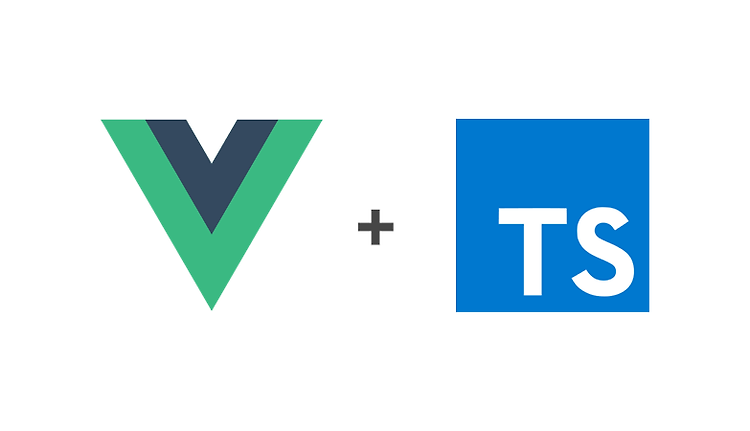
[Vue.js] Vue 3 + TypeScript #5 - 커스텀 타입 정의(Defining Custom Types)
하수도키
·2021. 3. 11. 13:36
2021.03.11 - [개발일기/Vue.js] - [Vue.js] Vue 3 + TypeScript #4 - 타입 기초(Type Fundamentals)
[Vue.js] Vue 3 + TypeScript #4 - 타입 기초(Type Fundamentals)
타입스크립트의 기본 개념 중 하나인 개발을 향상하는 데 사용하는 정적 타입 정의하는 방법을 살펴보자. 이번 포스팅에서는 타입스크립트에서 유용하고 자주 사용하는 일부 타입들을 살펴본
hasudoki.tistory.com
이전 포스팅 마지막에 보면 미리 정의 타입에 대한 아쉬운 점을 설명하면서 커스텀 타입에 대해 살펴봤다.
프로젝트가 커지고 요구사항이 많아질수록 커스텀 타입은 필수이다.
타입스크립트에서 커스텀 타입을 만드는 방법은 2가지이다.
type, interface를 사용한다.
type이란?(What is type?)
type은 간단하게 설명하면, 별칭(alias)을 지정하는 것이다.
데이터 형성(shaped)되어야 하는 다양한 방법을 참조하는 별칭?
type allows you to define an alias that refers to a specific way that the data should be shaped.
let buttonStyles: string = 'primary'
이전 포스팅에서 buttonStyles 변수 할당 코드를 보면 string으로 타입을 정의하는 게 한계였다.
type을 이용해 적합한 타입들만 사용할 수 있게 수정해보자.
type을 사용하는 방법(How to use type?)
변수 선언과 비슷하게 type도 선언해서 사용하면된다.
type buttonType = 'primary'
buttonType이라는 type을 선언했고 이 type은 'primary' 값을 포함한다.
사용하는 방법은 아래 코드와 같이 기존 정해진 type들과 동일하다.
let buttonStyles: buttonType = 'primary'
buttonType에 포함되지 않은 값들을 할당할 수 있다. 이럴 경우, 에러가 발생한다.
let buttonStyles: buttonType = 'secondary'
buttonType은 'primary'만 들어올 수 있게 작성되어 있기 때문이다.
만약 'primary, secondary' 값 모두 포함하는 타입을 정의하려면 어떻게 해야 될까?
다수의 값을 포함하는 type 정의하기(How to define multiple values?)
자바스크립트에서 다수의 값을 포함시키기 위해서 ||(union operator)를 사용했다.
if( a || b || c ) {...}
타입스크립트에 type을 정의할 때는 | 1개의 파이프를 사용한다.
type buttonType = 'primary' | 'secondary' | 'success' | 'danger'
// TypeScript will report an error because this doesn't exist in the type!
const errorBtnStyles: buttonType = 'error'
// This variable is type safe!
const dangerBtnStyles: buttonType = 'danger'
interface란? (What is an interface?)
inferface는 위에서 살펴본 type을 객체로 만든다고 생각하면 된다.
아래는 이전 포스팅에서 object type을 사용한 예제이다.
let person: {
name: string;
age: number;
activeAvenger: boolean;
powers: string[];
} = {
name: 'Peter Parker',
age: 20,
activeAvenger: true,
powers: ['wall-crawl', 'spider-sense']
}
위에 코드는 너무 복잡하지도 않지만, 코드가 너무 길고 장황한 느낌이 있다.
이걸 아래처럼 간단히 변경하는 과정을 살펴보자.
let person: Hero = {
name: 'Peter Parker',
age: 20,
activeAvenger: true,
powers: ['wall-crawl', 'spider-sense']
}
interface 정의하는 방법(How to define an interface?)
type과 같이 interface를 선언해야 한다.
interface Hero = {}
위에서 살펴본 person 객체 타입들을 Hero interface에 적용해보자.
interface Hero {
name: string;
age: number;
activeAvenger: boolean;
powers: string[];
}
이렇게 하면 interface 정의는 끝이다.
interface안에서 type사용이 가능할까?(Can you use type in an interface?)
Hero 인터페이스에 universe key를 추가해 Marvel 세계관인지 DC 세계관인지 구분하고 싶은 경우 바로 type을 사용한다.
type ComicUniverse = 'Marvel' | 'DC'
interface Hero {
name: string;
age: number;
activeAvenger: boolean;
powers: string[];
universe: ComicUniverse;
}
위와 같이 interface랑 type을 같이 사용할 수 있다.
결론
interface, type 2개 차이점이 항상 헷갈렸는데 여기서 간단하게 살펴보고 차이점을 이해할 수 있었다.
좀 더 자세한 차이점을 알고 싶으면 아래 링크를 참고
Type vs Interface, 언제 어떻게?
TypeScript에서 Type Alias와 Interface의 차이를 알아보기
medium.com
[Vue.js] Vue3 + TypeScript #6 마지막 커스텀 타입 정의하기(Type Assertion, data, props, computed, methods)
2021.03.05 - [개발일기/Vue.js] - [Vue.js] Vue3+Typescript #1 - Vue, TypeScript 사용하는 이유(Why Vue & TypeScript) 2021.03.11 - [개발일기/Vue.js] - [Vue.js] Vue 3 + TypeScript #5 - 커스텀 타입 정의(..
hasudoki.tistory.com